The idea is to come up with an approach to move the “daily maintenance” window if the current rule is going to take longer than anticipated and eventually collide with the maintenance window.
I choose to use the REST API to execute the business rule since it gives me the option to check other things while the rule is being executed.
Steps are as below:
- Retrieve the current daily maintenance time and convert it to date objects.
- Verify that the processing current rule is ‘x’ minutes or less from the existing daily maintenance time.
- Then, shift the daily maintenance window to the next time frame.
GET CURRENT MAINTENANCE WINDOW
I retrieved the details of the current maintenance window using the “Get” REST API method and changed them into system objects. I need to know both the current time and the maintenance time in order to calculate the difference in time. The system returns responses in UTC time zone. The code need to be adjusted if different time zones are involved.
Connection connection = operation.application.getConnection('localHost')
HttpResponse<String> jsonResponse = connection.get('/interop/rest/v1/services/dailymaintenance').header("Content-Type", 'application/json').asString()
def restResponseBody = new JsonSlurper().parseText(jsonResponse.body) as Map
if(restResponseBody.status == 0) {
Map<String, String> currentSettings = (restResponseBody?.items as List<Map<String, String>>)?.getAt(0)
println "Current Daily Maintenance Settings from the system :\n$currentSettings"
def(String maintenanceTime, String timeZone) = [ currentSettings.find{it.key == 'amwTime'}?.value, currentSettings.find{it.key == 'timeZone'}?.value ]
def currentDate = new Date()
def currentDateFormat = currentDate.format("yyyy-MM-dd HH:mm:ss")
def currentTime = new Date().parse("yyyy-MM-dd HH:mm:ss", currentDateFormat)
def maintTime = new Date().parse("yyyy-MM-dd HH:mm","${currentDateFormat.take(10)} ${maintenanceTime}")
println "Current Time : " + currentTime
long diffInSeconds = ( maintTime.getTime() - currentTime.getTime() ) / 1000 as long
UPDATE MAINTENANCE IF THE CURRENT TIME LESS THAN ‘X’ MINUTES
I have used 60 minute windows for my queries. If my current job executes within a 60-minute window of daily maintenance, I will shift the daily maintenance to the next window by calling the REST end points with next window parameter value.
if(diffInSeconds > 0 && diffInSeconds <= 3600 ) {
println "Maintenance Window in less than 1 hour. Moving the Maintenance Window"
Calendar calendar = Calendar.getInstance()
calendar.setTime(maintTime) ; calendar.add(Calendar.MINUTE, 60) ;
SimpleDateFormat dateFormat = new SimpleDateFormat("HH:mm")
String newMainTime = dateFormat.format(calendar.getTime())
println "Upadating Maintenance Time to $newMainTime"
jsonResponse = connection.put("/interop/rest/v1/services/dailymaintenance?StartTime=${newMainTime.take(2)}").header("Content-Type", 'application/json').asString()
restResponseBody = new JsonSlurper().parseText(jsonResponse.body) as Map
if(restResponseBody.status == 0) {
println "Daily Maintenance Window moved successfully."
}
} else {
println "Maintenance Window in not less than 1 hour. No changes applied"
}
I’d put everything in a method and call it while my current rule status is being evaluated. This method can be executed based on the preferred time interval.
RULE – EXECUTION
I was executing a sample rule and checking if the maintenance window was less than 1 hour or not.
WITHIN A 60 MINUTE TIME FRAME VALIDATION
DAILY MAINTENANCE WINDOW – BEFORE

BUSINESS RULE – EXECUTION STATUS

MASTER RULE – JOB CONSOLE LOG
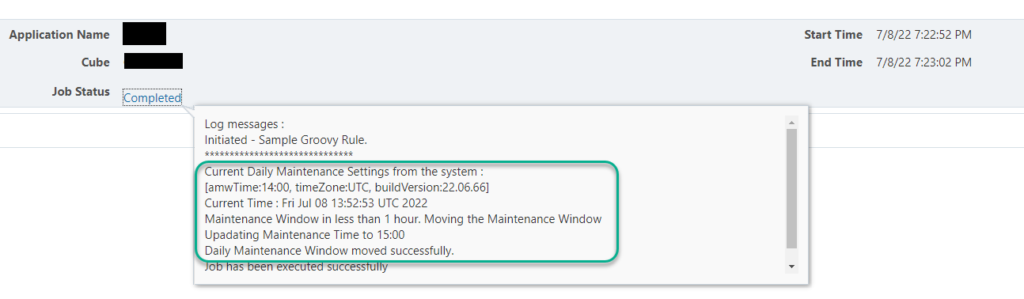
DAILY MAINTENANCE WINDOW – AFTER EXECUTION

OUTSIDE 60 MINUTE TIME FRAME VALIDATION
MASTER RULE – JOB CONSOLE LOG
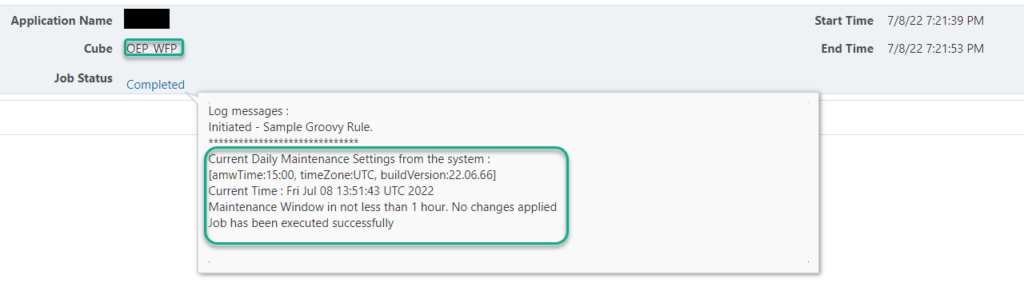
As you can see, the script is not updating the maintenance time because the maintenance time and the current time are both outside the 60-minute window. I hope this information is helpful.